Testing is critical in any system, especially asynchronous applications. It’s important to test each component in isolation, while carefully managing the communication between them. It is essential to ensure that everything works together seamlessly. For this reason, I would like to share with you the approach we take to testing in our Event-Driven system.
Testing with Rails Event Store - Practical Tips and Custom Solutions
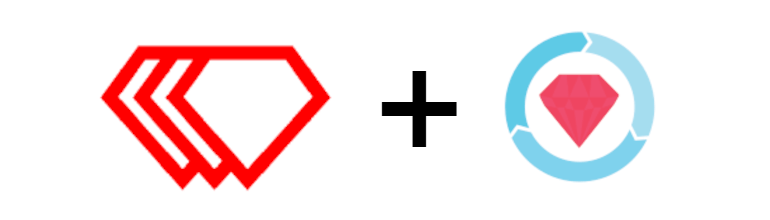